FRACTAL EXPERIMENTAL FIGURES
Mandelbrot-Lorenz Map
A Mandelbrot-Lorenz Map is a combination of a Mandelbrot figure and Lorenz calculations.
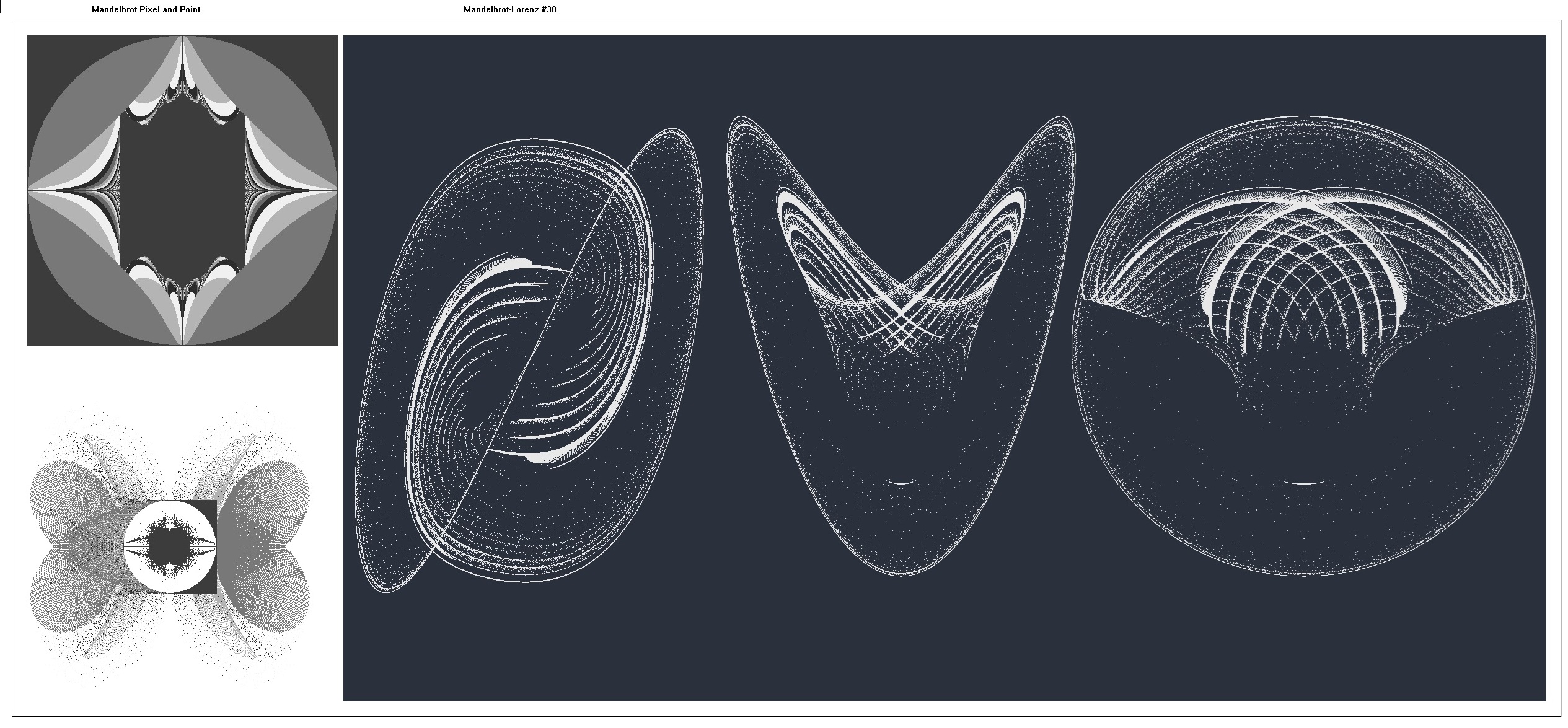
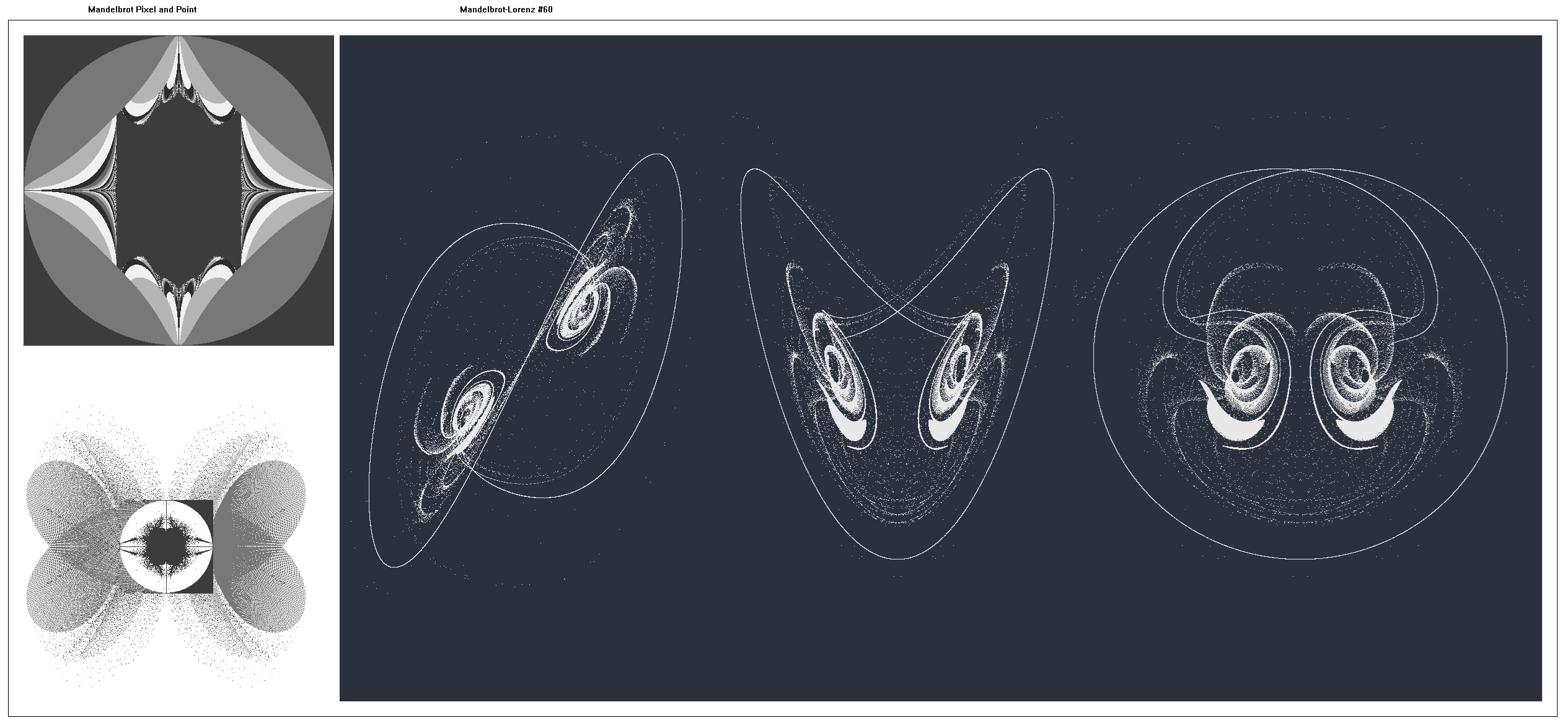
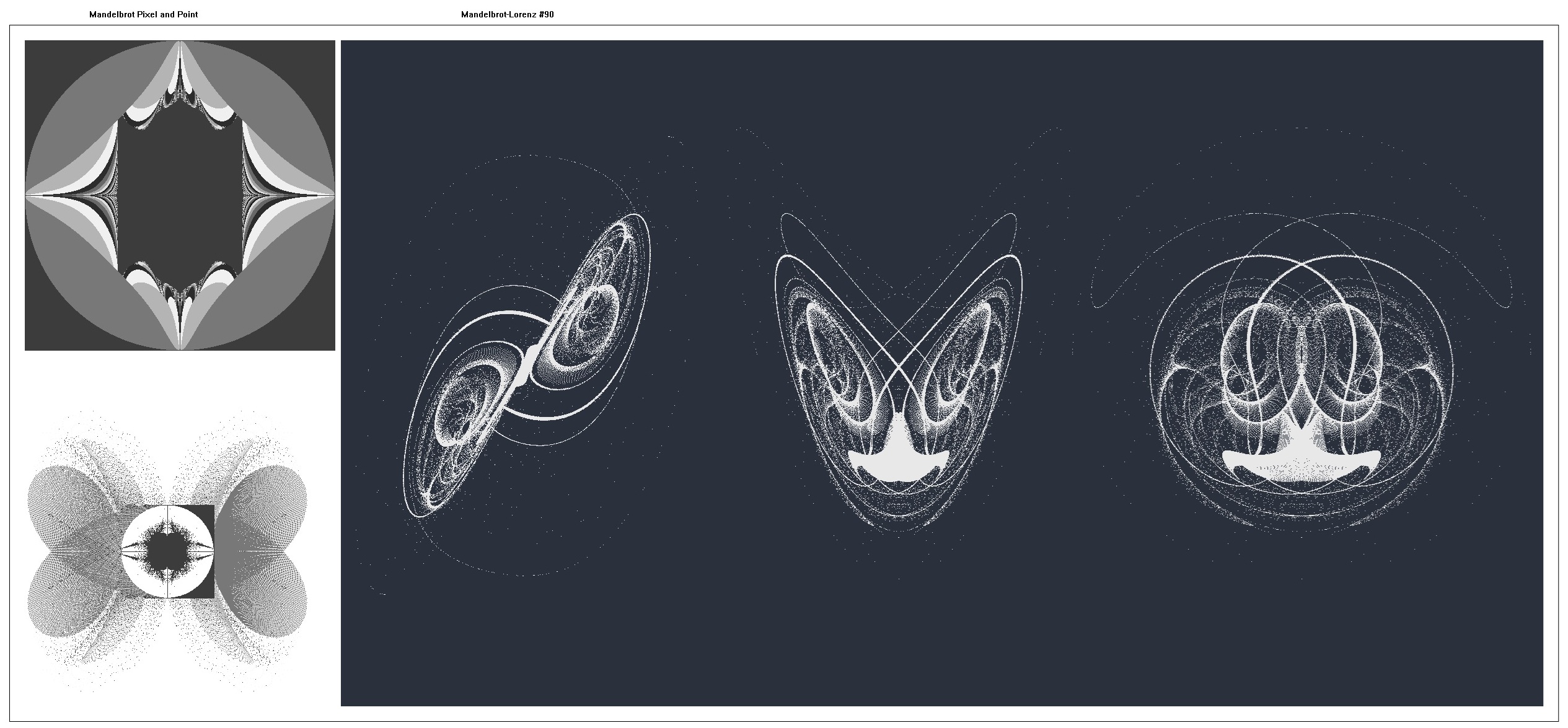
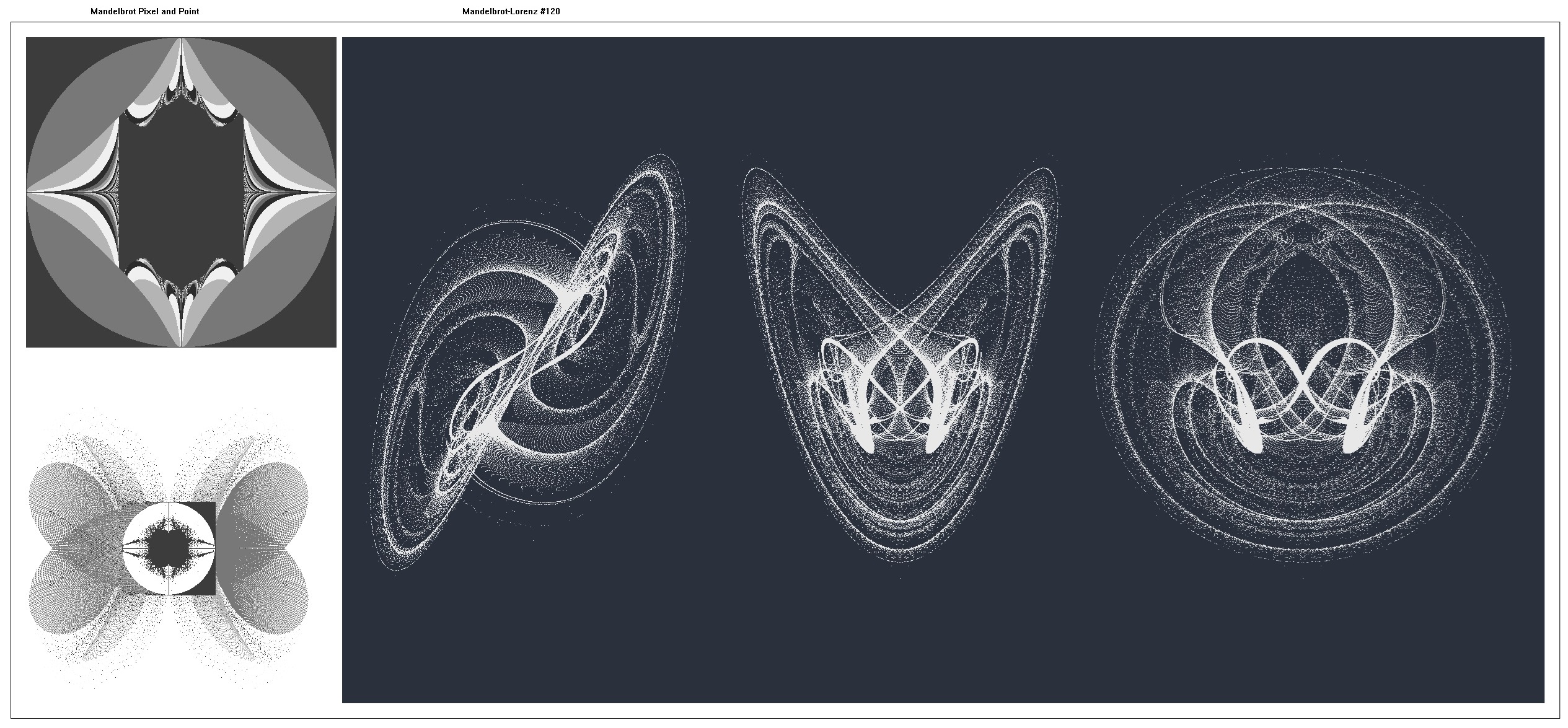
for (int i = 0; i ≤ 500; i++) { for (int j = 0; j ≤ 500; j++) { x = 0.0; y = 0.0; xs = -2.5 + (i / 100.0); ys = -2.5 + (j / 100.0); k = 0; do { xnew = x * y * y + xs; ynew = -x * x * y + ys; x = xnew; y = ynew; k = k + 1; } while ((k ≤ 64) && (x*x + y*y ≤ 6.25)); xarray[i][j] = x; yarray[i][j] = y; zarray[i][j] = k; PlotPixel(i, j, color); PlotPoint(x, y, color); } }
Two hundred iterations of the Mandelbrot-Lorenz Map (501 pixels x 501 pixels) were generated with the map plotted each ten interals. Here is map pseudocode:
dt=0.02, r=28.0, s=10.0, b=2.667 for (int m = 1; m ≤ 200; m++) { for (int i = 0; i ≤ 500; i++) { for (int j = 0; j ≤ 500; j++) { x = xarray[i][j]; y = yarray[i][j]; z = zarray[i][j]; dx = s * (y - x); dy = r * x - y - x * z; dz = x * y - b * z; xarray[i][j] = x = x + dx * dt; yarray[i][j] = y = y + dy * dt; zarray[i][j] = z = z + dz * dt; if (m % 10 == 0) { PlotPoint(x, y, color); PlotPoint(x, z, color); PlotPoint(y, z, color); } } } }
Resource for Lorenz calculations:
https://en.wikipedia.org/wiki/Lorenz_system
Mandelbrot Pixel Variations and Mandelbrot Point Variations
Chapter 1 presents Mandelbrot pixel variations and Mandelbrot point variations.
Here is pseudocode and here are standard Mandelbrot and Mandelbar figures where x*x+y*y ≤ 16.0 is presented in blue for each figure.
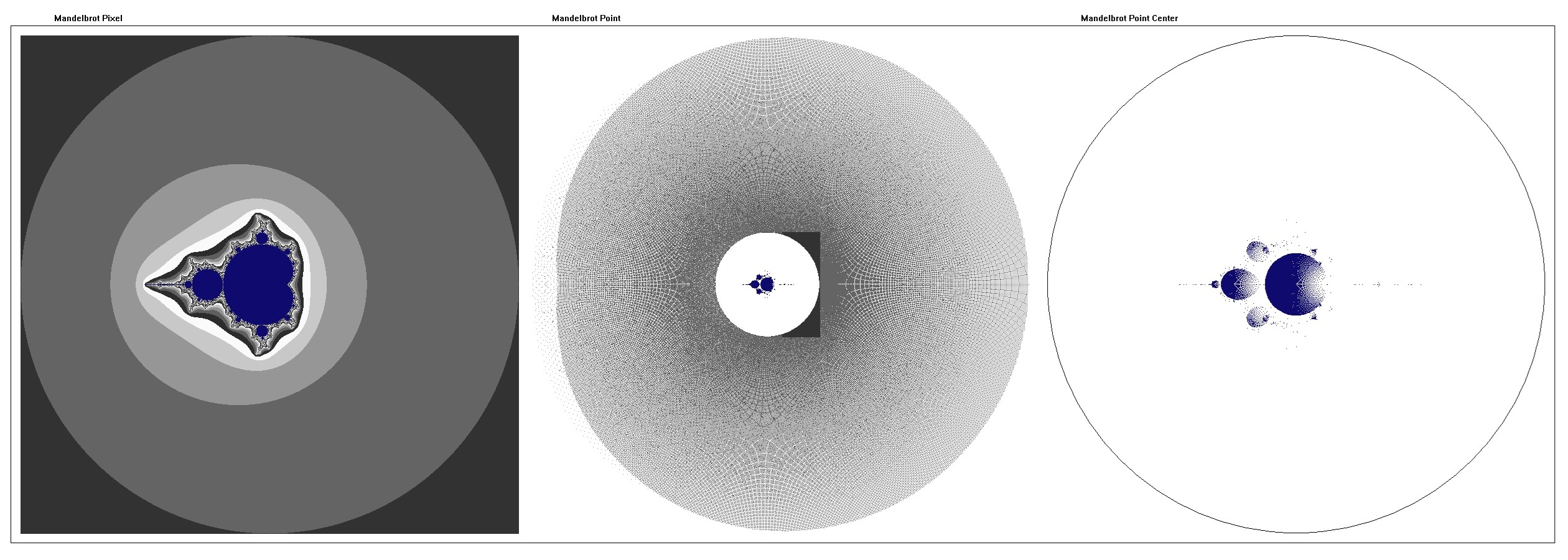
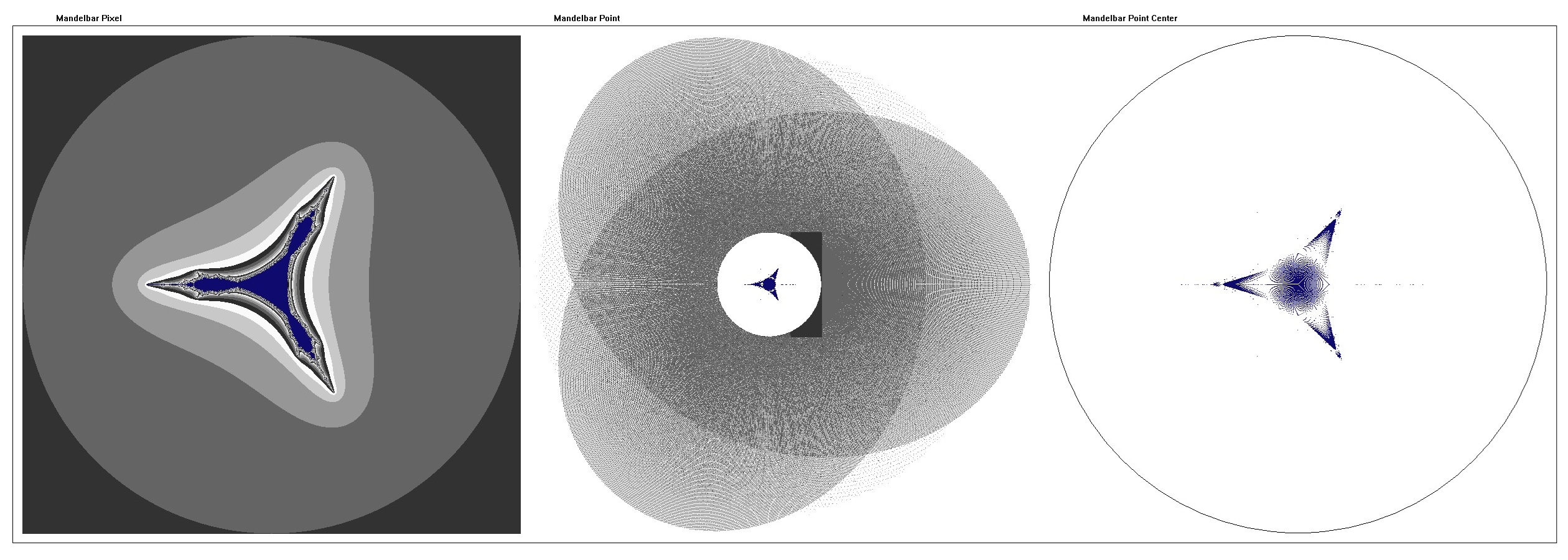
for (int i = 0; i ≤ 800; i++) { for (int j = 0; j ≤ 800; j++) { x = 0.0; y = 0.0; xs = -4.0 + (i / 100.0); ys = -4.0 + (j / 100.0); k = 0; do { k = k + 1; xnew = x * x - y * y + xs; ynew = 2.0 * x * y + ys; x = xnew; y = ynew; } while ((k ≤ kmax) && x*x+y*y ≤ 16.0); color = gray; if (x*x+y*y ≤ 16.0) color = blue; Plot respective pixels and points. } }
Mandelbrot and Mandelbar Tile
As shown in Chapter 2, here is a Mandelbrot tile and a Mandelbar tile.
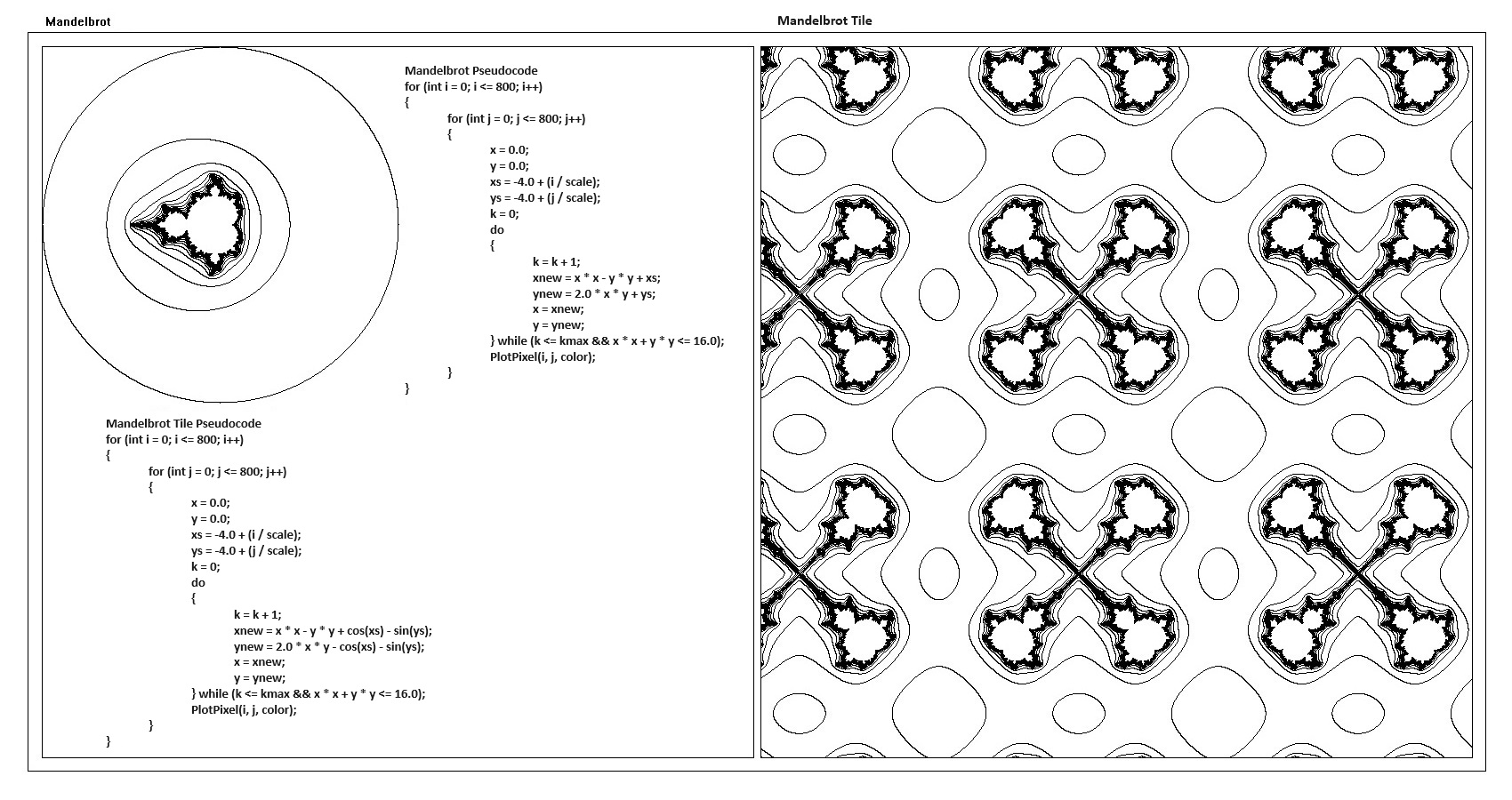
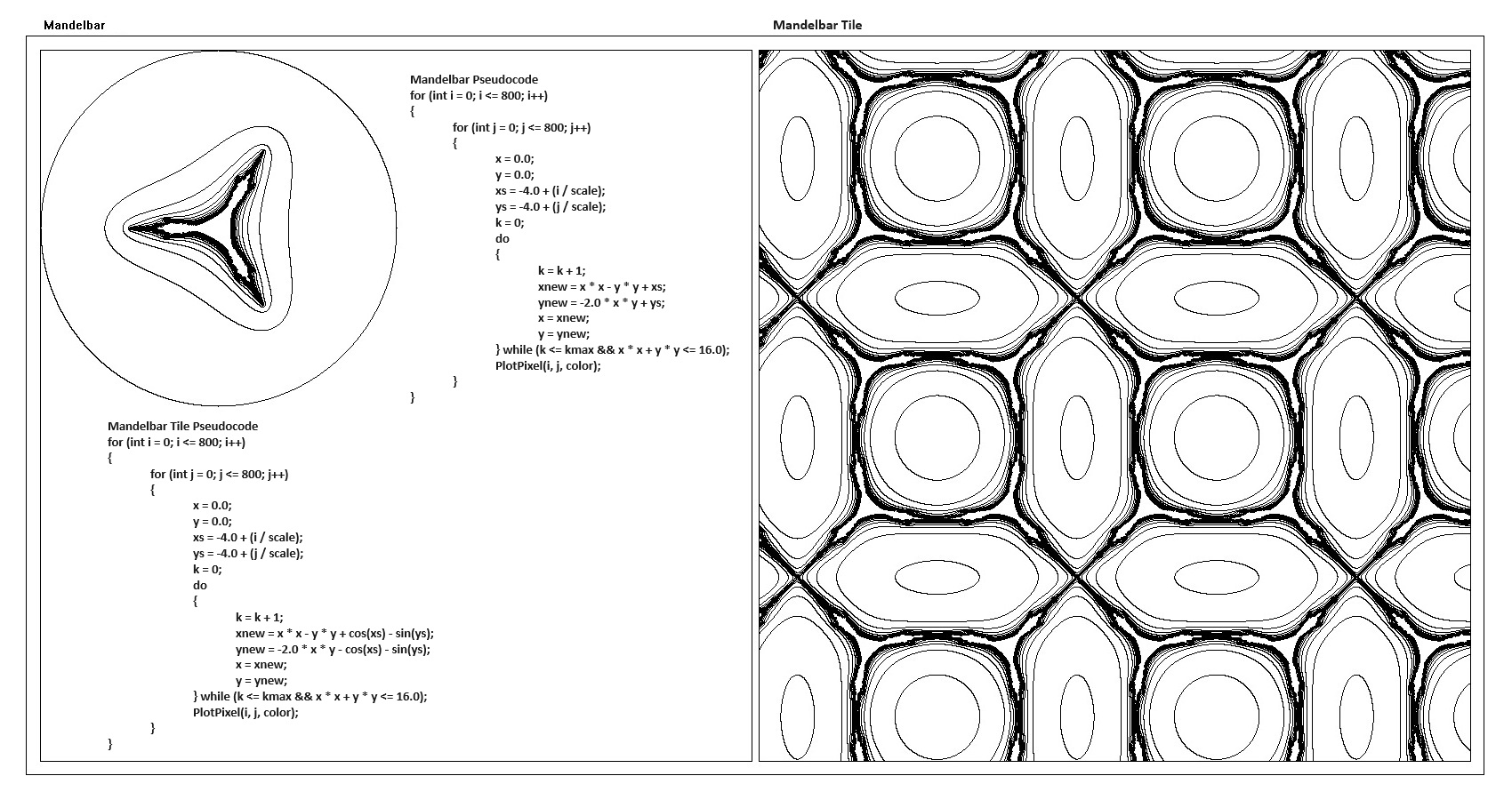
Quantum Figures
These figures show quantum values for a single value in the x-direction and a single value in the y-direction.
Both the basic Mandelbrot and basic Julia equations are used to create the figures. See Chapter 1 for further information on basic fractal figures.
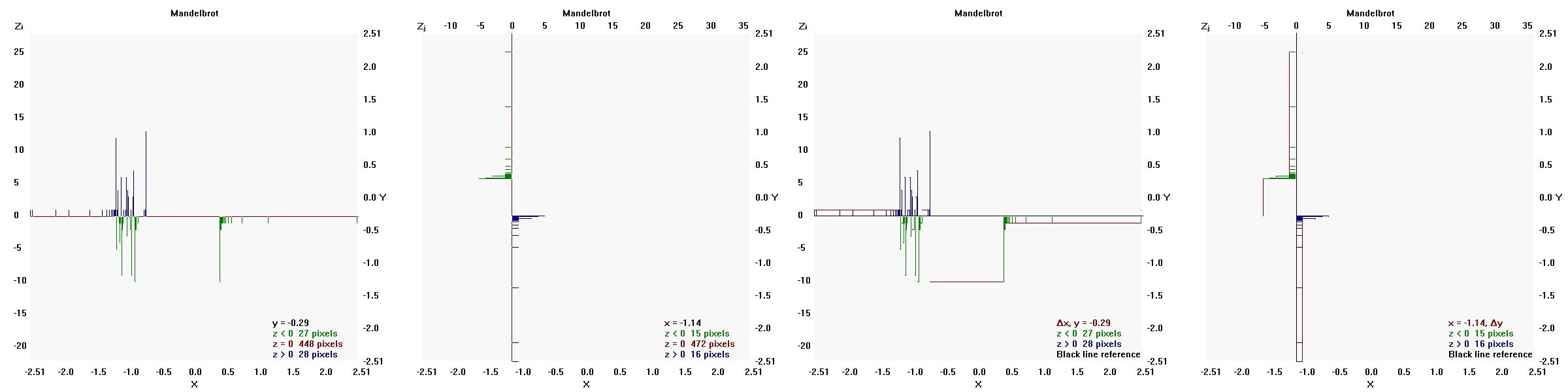
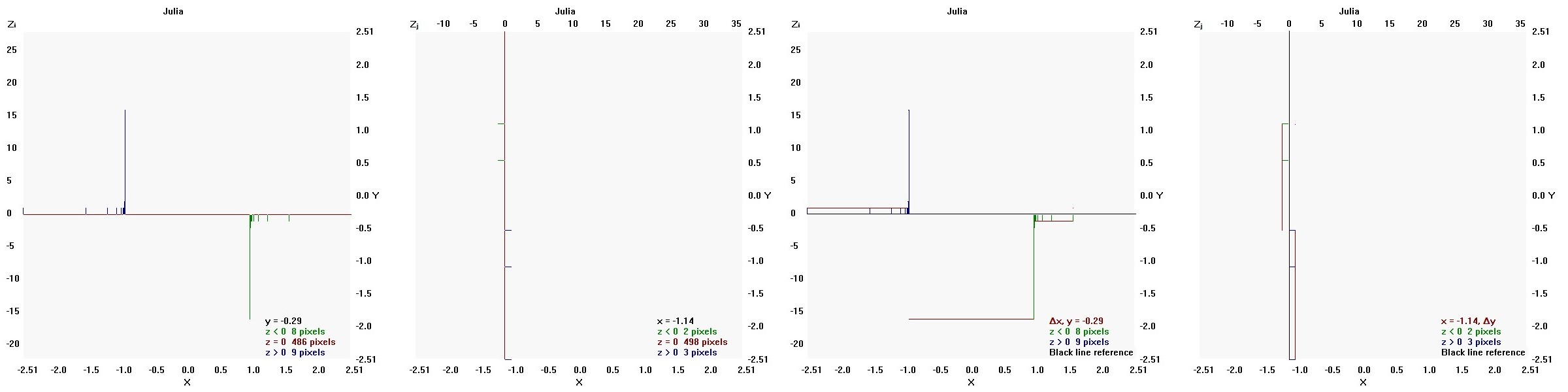